Imagine I have a website with user accounts. I want to authenticate users, so I need to know if the password they provide matches the one stored in my database. To protect the passwords from prying eyes, I want to obfuscate them, I decide to use AES in CBC mode to do so.
For instance, a counter is less likely to repeat (the chances of an IV colliding are relatively high due to the birthday problem). Do make sure that you are using a well seeded cryptographically secure random number generator. We want to store key and IV in Database and retrieve it for encryption/decryption. Key generator This page generates a wide range of encryption keys based on a pass phrase. Passphrase: aes-128-cbc: aes-128-cfb: aes-128-cfb1: aes-128-cfb8: aes-128-ecb: aes-128-ofb. New(key, AES.MODECFB,iv) crypt = generator.encrypt(padit(source)) cryptedStr = base64.b64encode(crypt) print cryptedStr generator = AES. Supported key lengths and IV lengths 1 You can use only hexadecimal characters, newlines, tabulators and new line characters if you decrypt a string. 2 Input text has an autodetect feature at your disposal. The autodetect detects for you if the content of Input text field is in form of a plain text or a hexadecimal string. You can turn off the feature by clicking on 'OFF'. Online interface to Advanced Encryption Standard (AES), a standard used by US government that uses a specific variant of Rijndael algorithm.
I know that I shouldn't use AES-CBC to do this, and probably should go with a hashing algorithm instead of an encryption one, but please, ignore this fact. It will help me understand things better if you do.
Aes Encryption Key Generator
When encrypting the user password to store it in my database using AES-CBC, do I need to use a Key, an IV and a Salt? Whatever I need to use, how would they be used exactly?
I'll give an example to better explain my issue:
A user with username 'bob' signs up with the password 'mypass'

I need to decide what is the safest way to obfuscate this password using AES-CBC.
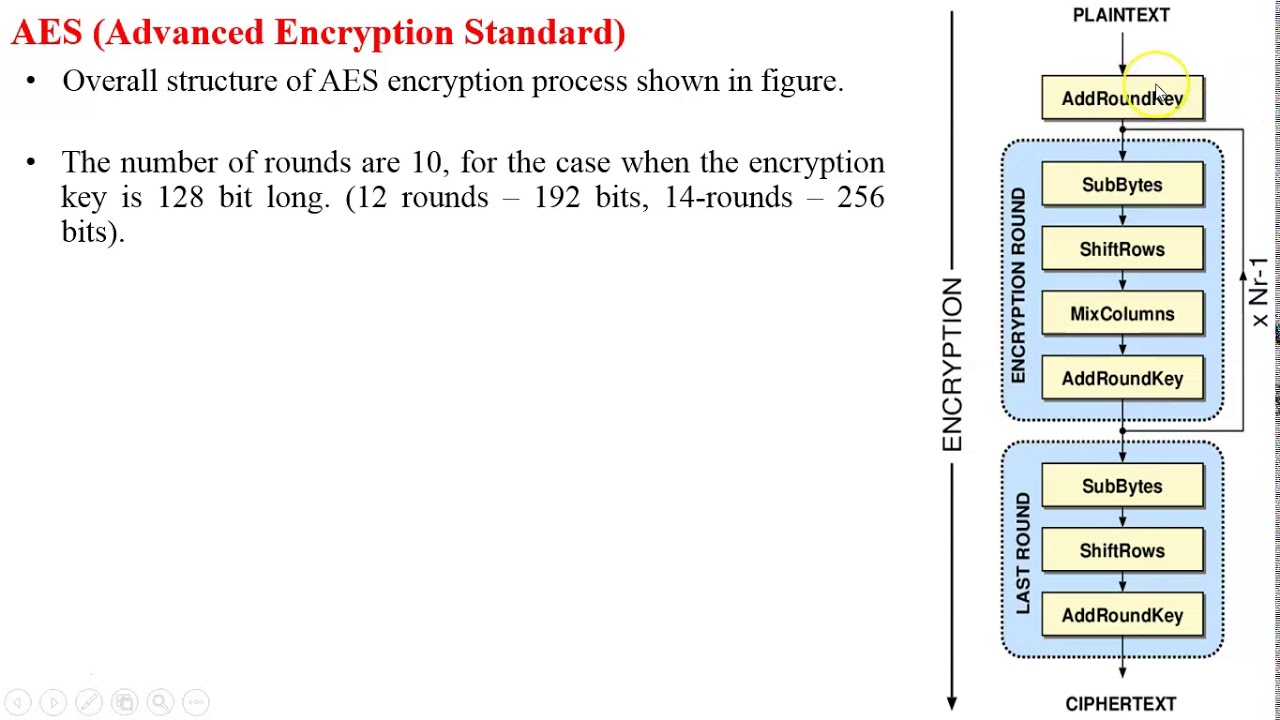
Right now I think to do this I need to generate a 16-byte salt using a CSPRNG[1]. Than I must prepend the salt with the user password: salt+'mypass'. Than I must encrypt salt+'mypass' using AES-CBC which I will use a hardcoded 256 bit key and a randomly generated IV of 16 byte.
Effectively I have: AesEncrypt(salt+'mypass', GenerateIv(), Key)
Given this example, I have a few points which confuses me.
Aes Key And Iv Generators
1) The random IV I am passing to AES will have the effect that no two 'mypass' gives the same ciphertext. Doesn't this make the salting redundant, because the point of the salt is to prevent two ciphertext of the 'mypass' to be identical?
2) Inversely, could it be that the IV is redundant, and I could just pass in the zero IV or a constant IV since I am salting my passwords?
3) Say the IV and the salt are not redundant, because somehow I read without really understanding that the IV is XORed but not the salt, making both useful. Is it enough for me to properly salt to simply prepend 'mypass' with the salt?
4) Am I somehow supposed to use the salt to derive the key that I will use for encrypting 'mypass' with AES? Or am I doing it right by simply using a hardcoded key?
5) What am I supposed to store in my database? Should the salt, the iv and the ciphertext all be stored in individual columns, or should they be somehow combined together, if so, is simple concatenation enough, or they must be combined with a special function?
6) Am I completely wrong and if so, what should I do instead?
Thank You.
[1] cryptographically secure pseudo-random number generator
PROGRAMMING INTERFACE
Internal state is maintained in an opaque structure that is returnedfrom the Init function. In ECB mode the state is not affected bythe input but for CBC mode some input dependent state is maintainedand may be reset by calling the Reset function with a newinitialization vector value.
Construct a new AES key schedule using the specified key data and thegiven initialization vector. The initialization vector is not usedwith ECB mode but is important for CBC mode.See MODES OF OPERATION for details about cipher modes.
Use a prepared key acquired by calling Init to encrypt theprovided data. The data argument should be a binary array that is amultiple of the AES block size of 16 bytes. The result is a binaryarray the same size as the input of encrypted data.
Decipher data using the key. Note that the same key may be used toencrypt and decrypt data provided that the initialization vector isreset appropriately for CBC mode.
Aes 128 Key Generator
Reset the initialization vector. This permits the programmer to re-usea key and avoid the cost of re-generating the key schedule where thesame key data is being used multiple times.
This should be called to clean up resources associated with Key.Once this function has been called the key may not be used again.